Archives
- Newer posts
- June 2025
- May 2025
- November 2024
- April 2024
- November 2023
- October 2023
- August 2023
- May 2023
- February 2023
- October 2022
- August 2022
- July 2022
- May 2022
- April 2022
- March 2022
- February 2022
- June 2020
- March 2020
- February 2020
- January 2020
- December 2019
- November 2019
- October 2019
- September 2019
- August 2019
- July 2019
- June 2019
- May 2019
- April 2019
- March 2019
- February 2019
- January 2019
- December 2018
- November 2018
- October 2018
- September 2018
- August 2018
- July 2018
- June 2018
- May 2018
- April 2018
- March 2018
- February 2018
- January 2018
- December 2017
- November 2017
- October 2017
- September 2017
- August 2017
- July 2017
- June 2017
- May 2017
- April 2017
- March 2017
- February 2017
- January 2017
- August 2016
- June 2016
- April 2016
- March 2016
- February 2016
- January 2016
- July 2015
- June 2015
- Older posts
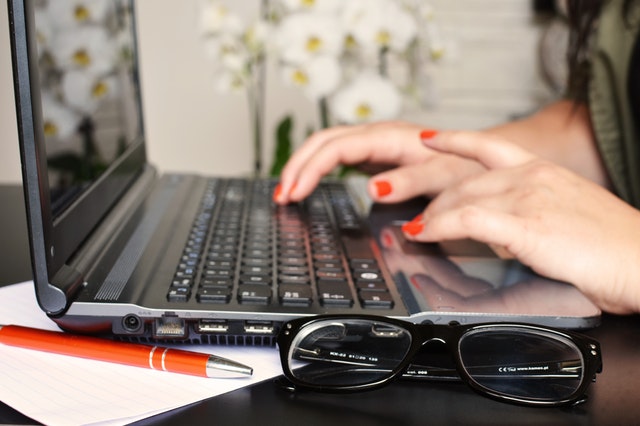
Guidelines for writing Selenium automation
Automated testing is the best way to increase the effectiveness, efficiency, and coverage of your web application. Automation can be helpful not only to debug the code but also to find the root cause of the defect by identifying the code section that is error-prone. This can be carried out through tests with Selenium WebDriver for web applications and Appium for native and mobile web applications.
Selenium is a testing framework to perform web application testing across various platforms like Windows, Mac, and Linux. Selenium supports only web-based applications that need a browser open. To execute the script on different browsers, we need the driver of that browser.
It supports various programming languages such as Java, PHP, C#, Python, Groovy, Ruby, and Perl for writing the test automation scripts. It offers recording and playback action features to write tests.
Below are the basic requirements for running a Selenium Java script.
Importing Packages
Two important packages that a tester needs to import when starting to code a script are:
- openqa.selenium.*- This package includes the WebDriver class needed to instantiate a new browser loaded with a specific driver.
- openqa.selenium.firefox.FirefoxDriver – FirefoxDriver class instantiates a Firefox-specific driver.
As the project would grow, it is evident and logical that we may need to introduce several other packages for more complex and distinct functionalities like database connectivity, manipulating external files, logging, assertions, accessing another class, taking browser screenshots etc.
Instantiating objects and variables
For Selenium automation, the very first line of code comes as:
WebDriver driver = new FirefoxDriver();
To initiate a driver, we create a reference variable for the WebDriver interface and instantiate it using FirefoxDriver class. It means that we are defining a reference variable (driver) whose type is an interface. Any object we assign to it must be an instance of class FireFoxDriver or any other drivers that implement that interface.
A default Firefox profile will be launched which means that no extensions and plugins would be loaded with the Firefox instance and that it runs in the safe mode.
Selenium WebDriver makes direct calls to the browser using each browser’s native support. How these direct calls are made, and the features they support depends on the browser you are using. Selenium standalone server uses this driver to start the browser of your choice. Normally to run webdriver, we just need a browser and a selenium server jar file. Selenium supports to run webdriver on the browsers by adding a .exe path of the driver server for the individual browsers.
Here are the code snippets for Firefox, Chrome and IE:
Firefox
System.setProperty(“webdriver.firefox.bin”,”F:\\Program Files(x86)\\Firefox\\Firefox.exe”);
WebDriver driver = new FirefoxDriver();
driver.navigate().to(“https://www.google.com”);
Chrome
System.setProperty(“webdriver.chrome.driver”,”G:\\Lib\\chromedriver.exe”);
WebDriver driver = new ChromeDriver();
driver.navigate().to(“https://www.google.com”);
Internet Explorer
System.setProperty(“webdriver.ie.driver”,”G:\\Lib\\internetexplorerdriver.exe”);
WebDriver driver = new InternetExplorerDriver();
driver.navigate().to(“https://www.google.com”);
Launching the Web browser
driver.get (baseURL);
To launch a fresh web browser instance the get() method is called on the WebDriver instance. The string character sequence passed as a parameter into the get() method redirects the launched web browser instance to the URL that you specify as its parameter.
Comparison between Expected and Actual Values:
Comparison code simply uses a basic if-else structure to compare the actual condition with the expected one.
if (actualCondition.contentEquals (expectedCondition)) {
System.out.printIn(“Success”);
} else {
System.out.printIn(“Fail”);
}
Terminating a Browser Session:
The “close()” method is used to close the browser window.
driver.close();
The scripts can be saved and re-run at any time. Hope this blog helps you in writing your first of many selenium test scripts.