Archives
- Newer posts
- April 2024
- November 2023
- October 2023
- August 2023
- May 2023
- February 2023
- October 2022
- August 2022
- July 2022
- May 2022
- April 2022
- March 2022
- February 2022
- June 2020
- March 2020
- February 2020
- January 2020
- December 2019
- November 2019
- October 2019
- September 2019
- August 2019
- July 2019
- June 2019
- May 2019
- April 2019
- March 2019
- February 2019
- January 2019
- December 2018
- November 2018
- October 2018
- September 2018
- August 2018
- July 2018
- June 2018
- May 2018
- April 2018
- March 2018
- February 2018
- January 2018
- December 2017
- November 2017
- October 2017
- September 2017
- August 2017
- July 2017
- June 2017
- May 2017
- April 2017
- March 2017
- February 2017
- January 2017
- August 2016
- June 2016
- April 2016
- March 2016
- February 2016
- January 2016
- July 2015
- June 2015
- Older posts
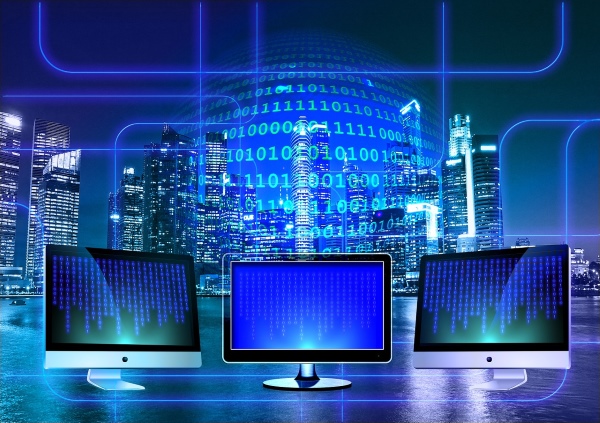
Repository Patterns
Reading and writing to the database is required by almost any system. Hence having an efficient method for communicating with the database is vital. Accessing the data in business logic can result in duplicated code, errors, weak typing, and inability to easily test the business logic in isolation. This is where repository patterns are very helpful.
Repository patterns maximize the amount of code that can be tested, allow for easy access to the data source, and improve the code’s maintainability and readability.
Repository pattern advantages are:
• Business logic can be unit tested without data access logic
• The database access code can be reused
• Database access code is centrally managed, making it easier to implement any database access policies.
A repository is a class defined for an entity, with all the operations possible on that specific entity.
For example, an entity Student will have operations like:
• void Insert(Student obj)
• void Update(Student obj)
• Student SelectById(string id)
An entity like Faculty will have operations like:
• void Insert(Faculty obj)
• void Update(Faculty obj)
• Faculty SelectById(string id)
This is an example for one repository per entity (non-generic). In this case, the two entities Student and Faculty, each have its own repository.
To further improve the efficiency, where there may be many entities with similar operations, we use Generic repositories. A generic repository is one that can be used for all entities. In the Generic repository interface, the operations will be defined using T instead of the entity.
For example, the operations will be:
• void Insert(T obj)
• void Update(T obj)
• T SelectById(string id)
This method can now be used for creating new students or creating new faculties since it is not entity-specific.
Benefits of Generic Repository Pattern:
• It reduces redundancy of code.
• It creates the possibility of less error.
• Easy to maintain the centralized data access logic.